Images
Inertia Table comes with a dedicated ImageColumn
, but you can also display images in other types of columns. For example, you may render an avatar image in front of a user's name.
The Image
class
On a column instance, like TextColumn
, you may call the image()
method to render an image in front of the text value. The image()
method accepts a callback that receives the Eloquent Model and an instance of the Image
class that you can use to configure the image.
use InertiaUI\Table\Image;
TextColumn::make('name')->image(function (User $user, Image $image) {
return $image->url($user->avatar_url);
});
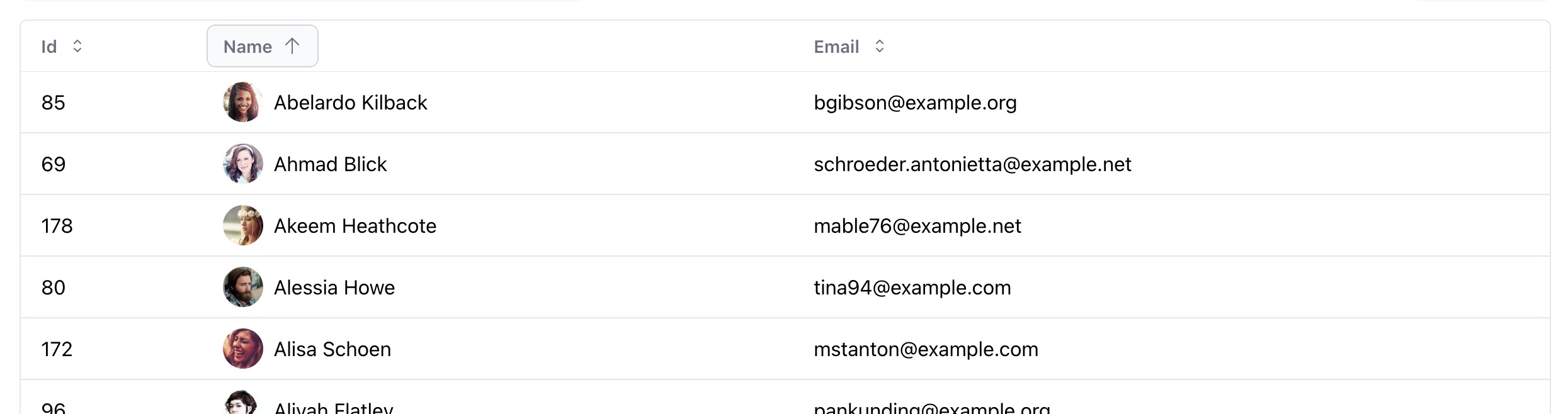
The Image
class has the following methods to set the URL:
$image
->url($user->avatar_url)
->to($user->avatar_url) // Alias of url()
->route('users.avatar', $user) // Equivalent to URL::route()
->signedRoute('users.avatar', $user) // Equivalent to URL::signedRoute()
->temporarySignedRoute('users.avatar', now()->addHour(), $user); // Equivalent to URL::temporarySignedRoute()
Styling the image
The Image
class allows you to style the image, such as making it rounded or adjusting its size. You can use the ImagePosition
and ImageSize
enums to set the image's position and size, or use helper methods for convenience.
use InertiaUI\Table\ImagePosition;
use InertiaUI\Table\ImageSize;
// Render the image fully rounded (applies the 'rounded-full' class)
$image->rounded();
// Set the image size using the ImageSize enum
$image->size(ImageSize::Large);
// Helper methods to set image size
$image->small(); // Uses the 'size-4' class
$image->medium(); // Uses the 'size-6' class
$image->large(); // Uses the 'size-8' class
$image->extraLarge(); // Uses the 'size-10' class
// Position the image at the start or end of the cell
$image->position(ImagePosition::Start);
// Helper methods to set image position
$image->start();
$image->end();
Instead of using size()
, you can also set dimensions manually with width()
, height()
, and dimensions()
:
$image->width(80);
$image->height(45);
$image->dimensions(80, 45); // Sets both width and height
You may also use the class()
method to add custom classes to the image:
$image->class('border border-red-500');
Ensure that your table's path is included in the content section of your Tailwind CSS configuration. Otherwise, Tailwind may purge these classes during the build process. For more details, refer to the content configuration documentation.
Alt and title attributes
You can use the alt()
and title()
methods to set the image attributes:
$image->alt($user->name);
$image->title($user->name);
Alternative syntax
Instead of passing a callback to the image()
method, you can directly pass the name of the Eloquent attribute containing the image URL:
TextColumn::make('name')->image('avatar_url');
To customize the image while using this syntax, pass a callback as the second argument:
TextColumn::make('name')->image('avatar_url', fn (Image $image) => $image->rounded());
Using icons
Instead of displaying an image, you can use an icon by calling the icon()
method on the Image
instance. Refer to the Icons documentation for details.
Columns\TextColumn::make('id')->image(function (User $user, Image $image) {
return $image->icon($user->company ? 'BuildingOfficeIcon' : 'UserIcon');
});
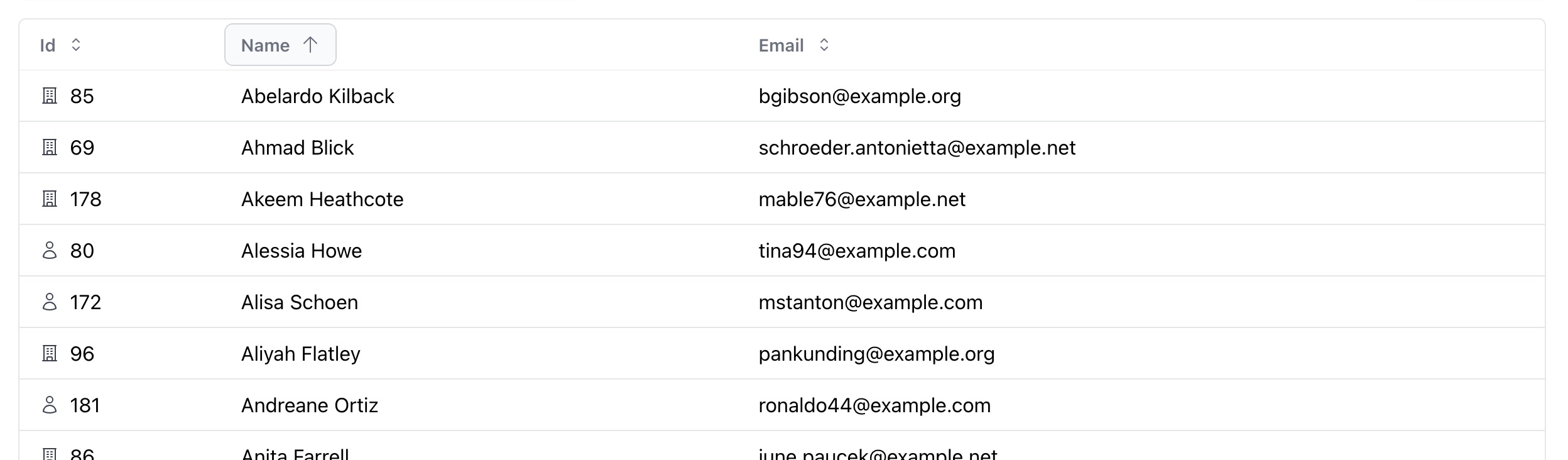
The ImageColumn
class
The ImageColumn
class is a specialized column that only renders images. Like other columns, you may call the image()
method to configure the image.
ImageColumn::make('avatar_url', header: '')->image(function (User $user, Image $image) {
return $image->rounded()->large();
});
Display multiple images
To display multiple images in a single cell, you may pass an array of URLs:
$image->url($user->friends->pluck('avatar_url')->all());
To limit the number of displayed images, use limit()
. In the example below, if the user has five friends, only the first three will be displayed, with a +2 indicator:
$image->url([...])->limit(3);
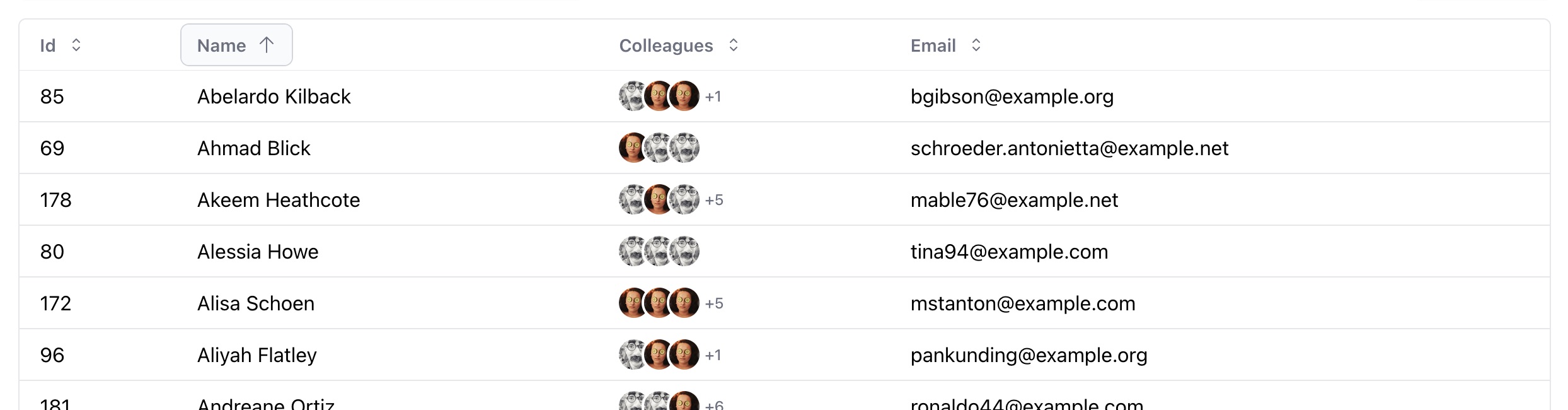
Fallback slot
If the image URL is empty and no icon is provided, you can define a fallback on the front-end. In Vue, use a dynamic slot name that corresponds to the column key (image-fallback(name)
). In React, pass an object to the imageFallback
prop.
<Table :resource="users">
<template #image-fallback(name)="{ item }">
<UserCircleIcon />
</template>
</Table>
<Table
resource={users}
imageFallback={{
name: ({ item }) => <UserCircleIcon />
}}
/>
Besides the item
prop, it also receives a column
, value
, image
, and table
prop. The image
prop represents the Image
class configuration.
Image slot
If you want to have full control how to render the image, you may use the image
slot, similar to the image-fallback
slot. It receives the same props.
<Table :resource="users">
<template #image(name)="{ item }">
<CustomImageComponent :src="item.avatar_url" />
</template>
</Table>
<Table
resource={users}
image={{
name: ({ item }) => <CustomImageComponent src={item.avatar} />
}}
/>